Class GraphUpdateScene
Extends
GraphModifier
Public
Helper class for easily updating graphs.
The GraphUpdateScene component is really easy to use. Create a new empty GameObject and add the component to it, it can be found in Components–>Pathfinding–>GraphUpdateScene.
When you have added the component, you should see something like the image below.
The region which the component will affect is defined by creating a polygon in the scene. If you make sure you have the Position tool enabled (top-left corner of the Unity window) you can shift+click in the scene view to add more points to the polygon. You can remove points using shift+alt+click. By clicking on the points you can bring up a positioning tool. You can also open the "points" array in the inspector to set each point's coordinates manually.
In the inspector there are a number of variables. The first one is named "Convex", it sets if the convex hull of the points should be calculated or if the polygon should be used as-is. Using the convex hull is faster when applying the changes to the graph, but with a non-convex polygon you can specify more complicated areas.
The next two variables, called "Apply On Start" and "Apply On Scan" determine when to apply the changes. If the object is in the scene from the beginning, both can be left on, it doesn't matter since the graph is also scanned at start. However if you instantiate it later in the game, you can make it apply it's setting directly, or wait until the next scan (if any). If the graph is rescanned, all GraphUpdateScene components which have the Apply On Scan variable toggled will apply their settings again to the graph since rescanning clears all previous changes.
You can also make it apply it's changes using scripting. GetComponent<GraphUpdateScene>().Apply ();
The above code will make it apply its changes to the graph (assuming a GraphUpdateScene component is attached to the same GameObject).
Next there is "Modify Walkability" and "Set Walkability" (which appears when "Modify Walkability" is toggled). If Modify Walkability is set, then all nodes inside the area will either be set to walkable or unwalkable depending on the value of the "Set Walkability" variable.
Penalty can also be applied to the nodes. A higher penalty (aka weight) makes the nodes harder to traverse so it will try to avoid those areas.
The tagging variables can be read more about on this page: Working with tags.
Note
The Y (up) axis of the transform that this component is attached to should be in the same direction as the up direction of the graph. So if you for example have a grid in the XY plane then the transform should have the rotation (-90,0,0).
Public Methods
void
DisableLegacyMode
()
Disables legacy mode if it is enabled.
Legacy mode is automatically enabled for components when upgrading from an earlier version than 3.8.6.
void
DrawGizmos
()
Draws some gizmos.
Bounds
GetBounds
()
Calculates the bounds for this component.
This is a relatively expensive operation, it needs to go through all points and run matrix multiplications.
void
InvertSettings
()
Inverts all invertable settings for this GUS.
Namely: penalty delta, walkability, tags.
Penalty delta will be changed to negative penalty delta.
setWalkability will be inverted.
setTag will be stored in a private variable, and the new value will be 0. When calling this function again, the saved value will be the new value.
Calling this function an even number of times without changing any settings in between will be identical to no change in settings.
void
LockToY
()
Lock all points to a specific Y value.
void
OnPostScan
()
Called right after all graphs have been scanned.
void
RecalcConvex
()
Recalculate convex hull.
Will not do anything if convex is disabled.
void
Start
()
Do some stuff at start.
Public Variables
bool
applyOnScan = true
Apply this graph update object whenever a graph is rescanned.
bool
applyOnStart = true
Apply this graph update object on start.
bool
convex = true
Use the convex hull of the points instead of the original polygon.
bool
legacyMode = false
Emulates behavior from before version 4.0.
float
minBoundsHeight = 1
Minumum height of the bounds of the resulting Graph Update Object.
Useful when all points are laid out on a plane but you still need a bounds with a height greater than zero since a zero height graph update object would usually result in no nodes being updated.
bool
modifyTag
Should the tags of the nodes be modified.
If enabled, set all nodes' tags to setTag
bool
modifyWalkability
If true, then all affected nodes will be made walkable or unwalkable according to setWalkability.
int
penaltyDelta
Penalty to add to nodes.
Usually you need quite large values, at least 1000-10000. A higher penalty means that agents will try to avoid those nodes more.
Be careful when setting negative values since if a node gets a negative penalty it will underflow and instead get really large. In most cases a warning will be logged if that happens.
Vector3[]
points
Points which define the region to update.
bool
resetPenaltyOnPhysics = true
Reset penalties to their initial values when updating grid graphs and updatePhysics is true.
If you want to keep old penalties even when you update the graph you may want to disable this option.
The images below shows two overlapping graph update objects, the right one happened to be applied before the left one. They both have updatePhysics = true and are set to increase the penalty of the nodes by some amount.
The first image shows the result when resetPenaltyOnPhysics is false. Both penalties are added correctly. 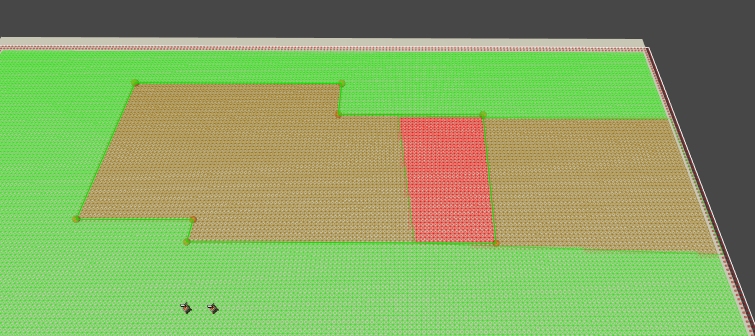
This second image shows when resetPenaltyOnPhysics is set to true. The first GUO is applied correctly, but then the second one (the left one) is applied and during its updating, it resets the penalties first and then adds penalty to the nodes. The result is that the penalties from both GUOs are not added together. The green patch in at the border is there because physics recalculation (recalculation of the position of the node, checking for obstacles etc.) affects a slightly larger area than the original GUO bounds because of the Grid Graph -> Collision Testing -> Diameter setting (it is enlarged by that value). So some extra nodes have their penalties reset.
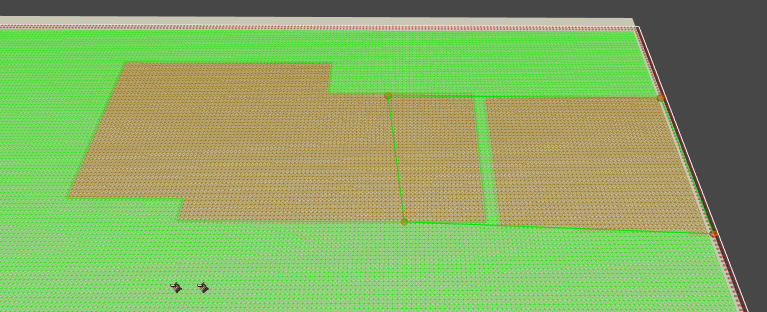
int
setTag
If modifyTag is enabled, set all nodes' tags to this value.
bool
setWalkability
Nodes will be made walkable or unwalkable according to this value if modifyWalkability is true.
bool
updateErosion = true
Update Erosion for GridGraphs.
When enabled, erosion will be recalculated for grid graphs after the GUO has been applied.
In the below image you can see the different effects you can get with the different values.
The first image shows the graph when no GUO has been applied. The blue box is not identified as an obstacle by the graph, the reason there are unwalkable nodes around it is because there is a height difference (nodes are placed on top of the box) so erosion will be applied (an erosion value of 2 is used in this graph). The orange box is identified as an obstacle, so the area of unwalkable nodes around it is a bit larger since both erosion and collision has made nodes unwalkable.
The GUO used simply sets walkability to true, i.e making all nodes walkable.
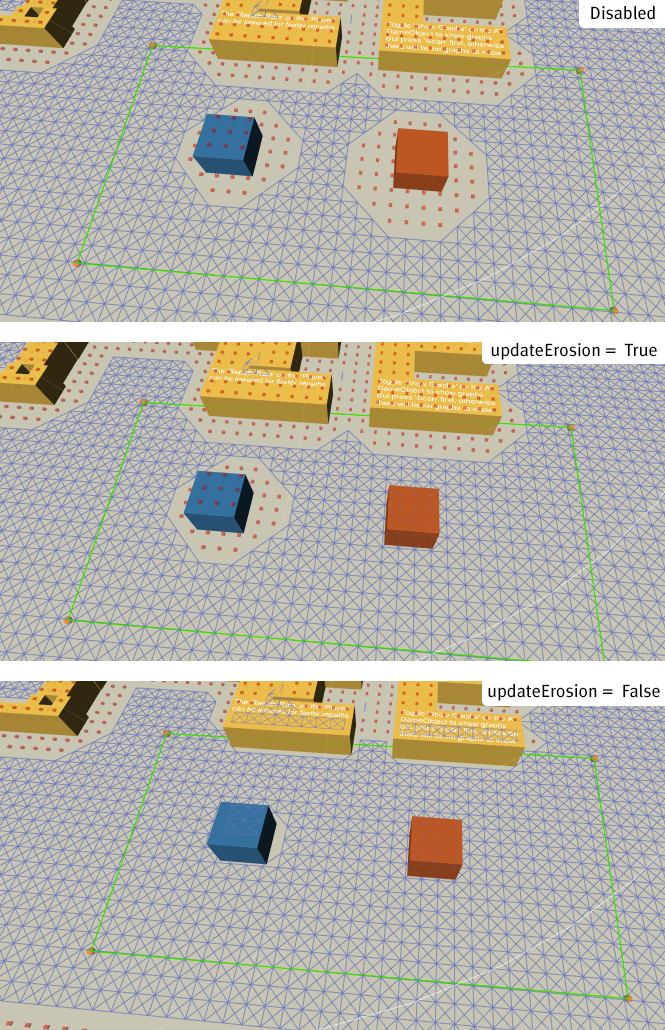
When updateErosion=True, the reason the blue box still has unwalkable nodes around it is because there is still a height difference so erosion will still be applied. The orange box on the other hand has no height difference and all nodes are set to walkable.
When updateErosion=False, all nodes walkability are simply set to be walkable in this example.
bool
updatePhysics
Update node's walkability and connectivity using physics functions.
For grid graphs, this will update the node's position and walkability exactly like when doing a scan of the graph. If enabled for grid graphs, modifyWalkability will be ignored.
For Point Graphs, this will recalculate all connections which passes through the bounds of the resulting Graph Update Object using raycasts (if enabled).
Inherited Public Members
EventType
PostScan= 1 << 0 |
|
PreScan= 1 << 1 |
|
LatePostScan= 1 << 2 |
|
PreUpdate= 1 << 3 |
|
PostUpdate= 1 << 4 |
|
PostCacheLoad= 1 << 5 |
|
PostUpdateBeforeAreaRecalculation= 1 << 6 |
|
PostGraphLoad= 1 << 7 |
|
GraphModifier event type.
void
OnGraphsPostUpdate
()
Called after graphs have been updated using GraphUpdateObjects or navmesh cutting.
This is among other times called after graphs have been scanned, updated using GraphUpdateObjects, navmesh cuts, or GraphUpdateScene components.
Area recalculations (see HierarchicalGraph) have been done at this stage so things like PathUtilities.IsPathPossible will work.
Use OnGraphsPostUpdateBeforeAreaRecalculation instead if you are modifying the graph in any way, especially connections and walkability. This is because if you do this then area recalculations
void
OnGraphsPostUpdateBeforeAreaRecalculation
()
Called after graphs have been updated.
This is among other times called after graphs have been scanned, updated using GraphUpdateObjects, navmesh cuts, or GraphUpdateScene components.
Use this if you are modifying any graph connections or walkability.
void
OnGraphsPreUpdate
()
Called before graphs are updated using GraphUpdateObjects.
void
OnLatePostScan
()
Called at the end of the scanning procedure.
This is the absolute last thing done by Scan.
void
OnPostCacheLoad
()
Called after cached graphs have been loaded.
When using cached startup, this event is analogous to OnLatePostScan and implementing scripts should do roughly the same thing for both events.
void
OnPostGraphLoad
()
Called after a graph has been deserialized and loaded.
Note
The graph may not have had any valid node data, it might just contain the graph settings.
This will be called often outside of play mode. Make sure to check Application.isPlaying if appropriate.
void
OnPreScan
()
Called right before graphs are going to be scanned.
void
TriggerEvent
(
)
Triggers an event for all active graph modifiers.
Private/Protected Members
Vector3[]
convexPoints
Private cached convex hull of the points.
bool
firstApplied
Has apply been called yet.
Used to prevent applying twice when both applyOnScan and applyOnStart are enabled
List<T>
GetModifiersOfType< T >
()
bool
legacyUseWorldSpace
Use world space for coordinates.
If true, the shape will not follow when moving around the transform.
void
OnDisable
()
Removes this modifier from list of active modifiers.
void
OnEnable
()
Adds this modifier to list of active modifiers.
int
OnUpgradeSerializedData
(
int | version | |
bool | unityThread | |
)
Handle serialization backwards compatibility.
void
Reset
()
Handle serialization backwards compatibility.
int
serializedVersion = 0
void
ToggleUseWorldSpace
()
Switches between using world space and using local space.
ulong
uniqueID
Unique persistent ID for this component, used for serialization.
Dictionary<ulong,GraphModifier>
usedIDs = new Dictionary<ulong, GraphModifier>()
Maps persistent IDs to the component that uses it.