int
CountNodes
()
Number of nodes in the graph.
Note that this is, unless the graph type has overriden it, an O(n) operation.
This is an O(1) operation for grid graphs and point graphs. For layered grid graphs it is an O(n) operation.
void
GetNodes
(
)
Calls a delegate with all nodes in the graph.
This is the primary way of iterating through all nodes in a graph.
Do not change the graph structure inside the delegate.
var gg = AstarPath.active.data.gridGraph;
gg.GetNodes(node => {
// Here is a node
Debug.Log("I found a node at position " + (Vector3)node.position);
});
If you want to store all nodes in a list you can do this
var gg = AstarPath.active.data.gridGraph;
List<GraphNode> nodes = new List<GraphNode>();
gg.GetNodes((System.Action<GraphNode>)nodes.Add);
void
CalculateConnectionsForCellAndNeighbours
(
)
Calculates the grid connections for a cell as well as its neighbours.
This is a useful utility function if you want to modify the walkability of a single node in the graph.
AstarPath.active.AddWorkItem(ctx => {
var grid = AstarPath.active.data.gridGraph;
int x = 5;
int z = 7;
// Mark a single node as unwalkable
grid.GetNode(x, z).Walkable = false;
// Recalculate the connections for that node as well as its neighbours
grid.CalculateConnectionsForCellAndNeighbours(x, z);
});
Warning
If you are recalculating connections for a lot of nodes at the same time, use RecalculateConnectionsInRegion instead, since that will be much faster.
bool
Linecast
(
)
Returns if there is an obstacle between from and to on the graph.
This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
var gg = AstarPath.active.data.gridGraph;
bool anyObstaclesInTheWay = gg.Linecast(transform.position, enemy.position);
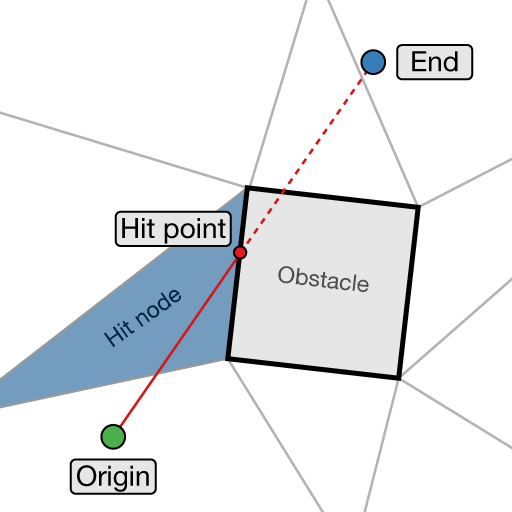
Edge cases are handled as follows:
Shared edges and corners between walkable and unwalkable nodes are treated as walkable (so for example if the linecast just touches a corner of an unwalkable node, this is allowed).
If the linecast starts outside the graph, a hit is returned at from.
If the linecast starts inside the graph, but the end is outside of it, a hit is returned at the point where it exits the graph (unless there are any other hits before that).
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
Vector3 | from | Point to linecast from |
Vector3 | to | Point to linecast to |
outGraphHitInfo | hit | Contains info on what was hit, see GraphHitInfo. |
List<GraphNode> | trace=null | If a list is passed, then it will be filled with all nodes the linecast traverses |
System.Func<GraphNode, bool> | filter=null | If not null then the delegate will be called for each node and if it returns false the node will be treated as unwalkable and a hit will be returned. Note that unwalkable nodes are always treated as unwalkable regardless of what this filter returns. |
)
Returns if there is an obstacle between from and to on the graph.
This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
Edge cases are handled as follows:
Shared edges and corners between walkable and unwalkable nodes are treated as walkable (so for example if the linecast just touches a corner of an unwalkable node, this is allowed).
If the linecast starts outside the graph, a hit is returned at from.
If the linecast starts inside the graph, but the end is outside of it, a hit is returned at the point where it exits the graph (unless there are any other hits before that).
var gg = AstarPath.active.data.gridGraph;
bool anyObstaclesInTheWay = gg.Linecast(transform.position, enemy.position);
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
GridNodeBase | fromNode | Node to start from. |
GridNodeBase | toNode | Node to try to reach using a straight line. |
System.Func<GraphNode, bool> | filter=null | If not null then the delegate will be called for each node and if it returns false the node will be treated as unwalkable and a hit will be returned. Note that unwalkable nodes are always treated as unwalkable regardless of what this filter returns. |
)
Returns if there is an obstacle between the two nodes on the graph.
This method is very similar to the other Linecast methods however it is a bit faster due to not having to look up which node is closest to a particular input point.
var gg = AstarPath.active.data.gridGraph;
var node1 = gg.GetNode(2, 3);
var node2 = gg.GetNode(5, 7);
bool anyObstaclesInTheWay = gg.Linecast(node1, node2);
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
Vector3 | from | Point to linecast from |
Vector3 | to | Point to linecast to |
outGridHitInfo | hit | Contains info on what was hit, see GridHitInfo |
List<GraphNode> | trace=null | If a list is passed, then it will be filled with all nodes the linecast traverses |
System.Func<GraphNode, bool> | filter=null | If not null then the delegate will be called for each node and if it returns false the node will be treated as unwalkable and a hit will be returned. Note that unwalkable nodes are always treated as unwalkable regardless of what this filter returns. |
)
Returns if there is an obstacle between from and to on the graph.
This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
Note
This overload outputs a hit of type GridHitInfo instead of GraphHitInfo. It's a bit faster to calculate this output and it can be useful for some grid-specific algorithms.
Edge cases are handled as follows:
Shared edges and corners between walkable and unwalkable nodes are treated as walkable (so for example if the linecast just touches a corner of an unwalkable node, this is allowed).
If the linecast starts outside the graph, a hit is returned at from.
If the linecast starts inside the graph, but the end is outside of it, a hit is returned at the point where it exits the graph (unless there are any other hits before that).
var gg = AstarPath.active.data.gridGraph;
bool anyObstaclesInTheWay = gg.Linecast(transform.position, enemy.position);
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
GridNodeBase | fromNode | Node to start from. |
Vector2 | normalizedFromPoint | Where in the start node to start. This is a normalized value so each component must be in the range 0 to 1 (inclusive). |
GridNodeBase | toNode | Node to try to reach using a straight line. |
Vector2 | normalizedToPoint | Where in the end node to end. This is a normalized value so each component must be in the range 0 to 1 (inclusive). |
outGridHitInfo | hit | |
List<GraphNode> | trace=null | |
System.Func<GraphNode, bool> | filter=null | If not null then the delegate will be called for each node and if it returns false the node will be treated as unwalkable and a hit will be returned. Note that unwalkable nodes are always treated as unwalkable regardless of what this filter returns. |
bool | continuePastEnd=false | If true, the linecast will continue past the end point in the same direction until it hits something. |
)
Returns if there is an obstacle between the two nodes on the graph.
This method is very similar to the other Linecast methods but it gives some extra control, in particular when the start/end points are at node corners instead of inside nodes.
Shared edges and corners between walkable and unwalkable nodes are treated as walkable. So for example if the linecast just touches a corner of an unwalkable node, this is allowed.
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
float
NearestNodeDistanceSqrLowerBound
(
Vector3 | position | The position to check from |
NNConstraint | constraint | A constraint on which nodes are valid. This may or may not be used by the function. You may pass null if you consider all nodes valid. |
)
Lower bound on the squared distance from the given point to the closest node in this graph.
This is used to speed up searching for the closest node when there is more than one graph in the scene, by checking the graphs in order of increasing estimated distance to the point.
Implementors may return 0 at all times if it is hard to come up with a good lower bound. It is more important that this function is fast than that it is accurate.
void
RecalculateConnectionsInRegion
(
)
Recalculates node connections for all nodes in a given region of the grid.
This is used if you have manually changed the walkability, or other parameters, of some grid nodes, and you need their connections to be recalculated. If you are changing the connections themselves, you should use the GraphNode.AddConnection and GraphNode.RemoveConnection functions instead.
Typically you do not change walkability manually. Instead you can use for example a GraphUpdateObject.
Warning
This method has some constant overhead, so if you are making several changes to the graph, it is best to batch these updates and only make a single call to this method.
Note
This will not take into account any grid graph rules that modify connections. So if you have any of those added to the grid graph, you probably want to do a regular graph update instead.
void
RelocateNodes
(
)
Moves the nodes in this graph.
Multiplies all node positions by deltaMatrix.
For example if you want to move all your nodes in e.g a point graph 10 units along the X axis from the initial position var graph = AstarPath.data.pointGraph;
var m = Matrix4x4.TRS (new Vector3(10,0,0), Quaternion.identity, Vector3.one);
graph.RelocateNodes (m);
Note
For grid graphs, navmesh graphs and recast graphs it is recommended to use their custom overloads of the RelocateNodes method which take parameters for e.g center and nodeSize (and additional parameters) instead since they are both easier to use and are less likely to mess up pathfinding.
Warning
This method is lossy for PointGraphs, so calling it many times may cause node positions to lose precision. For example if you set the scale to 0 in one call then all nodes will be scaled/moved to the same point and you will not be able to recover their original positions. The same thing happens for other - less extreme - values as well, but to a lesser degree.
void
RelocateNodes
(
Vector3 | center | |
Quaternion | rotation | |
float | nodeSize | |
float | aspectRatio=1 | |
float | isometricAngle=0 | |
)
Relocate the grid graph using new settings.
This will move all nodes in the graph to new positions which matches the new settings.
// Move the graph to the origin, with no rotation, and with a node size of 1.0
var gg = AstarPath.active.data.gridGraph;
gg.RelocateNodes(center: Vector3.zero, rotation: Quaternion.identity, nodeSize: 1.0f);
float
isometricAngle
Angle in degrees to use for the isometric projection.
If you are making a 2D isometric game, you may want to use this parameter to adjust the layout of the graph to match your game. This will essentially scale the graph along one of its diagonals to produce something like this:
A perspective view of an isometric graph. 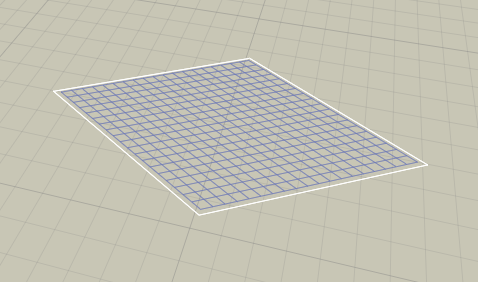
A top down view of an isometric graph. Note that the graph is entirely 2D, there is no perspective in this image. 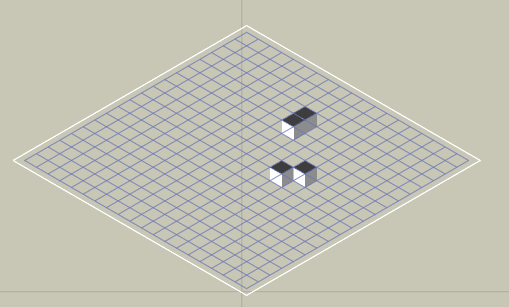
For commonly used values see StandardIsometricAngle and StandardDimetricAngle.
Usually the angle that you want to use is either 30 degrees (alternatively 90-30 = 60 degrees) or atan(1/sqrt(2)) which is approximately 35.264 degrees (alternatively 90 - 35.264 = 54.736 degrees). You might also want to rotate the graph plus or minus 45 degrees around the Y axis to get the oritientation required for your game.
You can read more about it on the wikipedia page linked below.
bool
maxStepUsesSlope = true
Take the slope into account for maxClimb.
When this is enabled the normals of the terrain will be used to make more accurate estimates of how large the steps are between adjacent nodes.
When this is disabled then calculated step between two nodes is their y coordinate difference. This may be inaccurate, especially at the start of steep slopes.
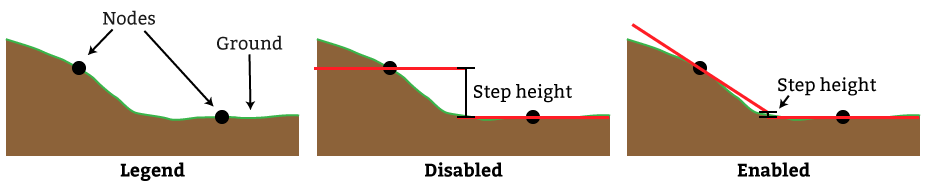
In the image below you can see an example of what happens near a ramp. In the topmost image the ramp is not connected with the rest of the graph which is obviously not what we want. In the middle image an attempt has been made to raise the max step height while keeping maxStepUsesSlope disabled. However this causes too many connections to be added. The agent should not be able to go up the ramp from the side. Finally in the bottommost image the maxStepHeight has been restored to the original value but maxStepUsesSlope has been enabled. This configuration handles the ramp in a much smarter way. Note that all the values in the image are just example values, they may be different for your scene. 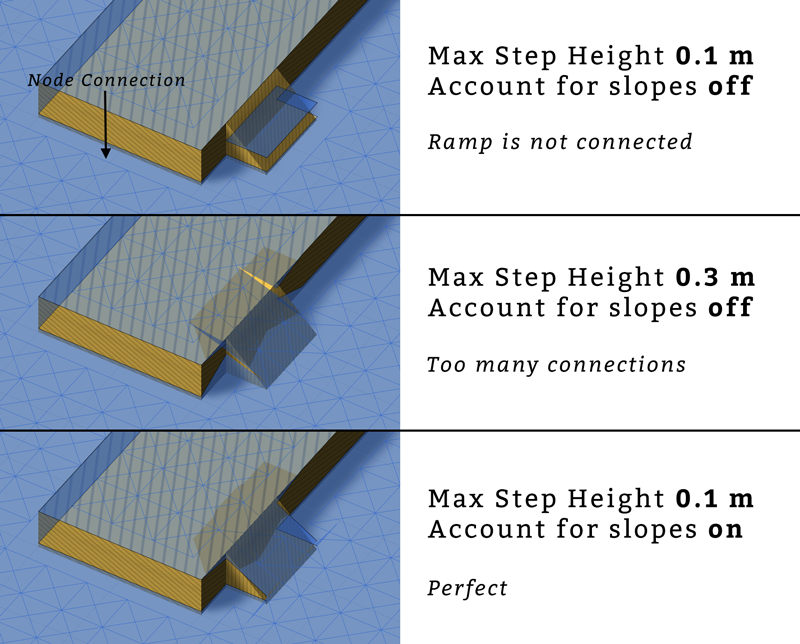
bool
ClipLineSegmentToBounds
(
)
Clips a line segment in graph space to the graph bounds.
That is (0,0,0) is the bottom left corner of the graph and (width,0,depth) is the top right corner. The first node is placed at (0.5,y,0.5). One unit distance is the same as nodeSize.
Returns false if the line segment does not intersect the graph at all.
void
PostDeserialization
(
)
Called after all deserialization has been done for all graphs.
Can be used to set up more graph data which is not serialized