Public
Generates a grid of nodes.
The GridGraph does exactly what the name implies, generates nodes in a grid pattern.
Grid graphs suit well to when you already have a grid based world. Features:
You can update the graph during runtime (good for e.g Tower Defence or RTS games)
Throw any scene at it, with minimal configurations you can get a good graph from it.
Supports raycast and the funnel algorithm
Predictable pattern
Can apply penalty and walkability values from a supplied image
Perfect for terrain worlds since it can make areas unwalkable depending on the slope
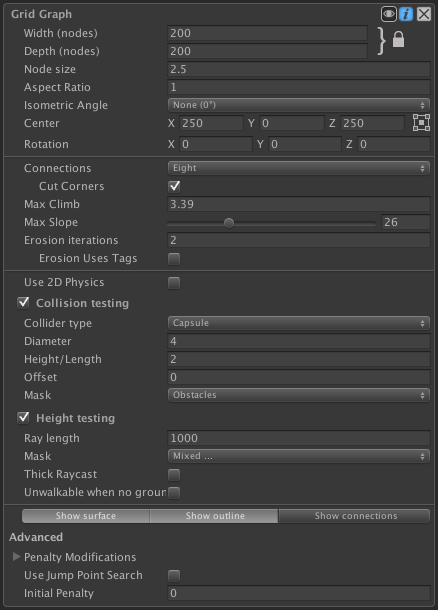
The The Snap Size button snaps the internal size of the graph to exactly contain the current number of nodes, i.e not contain 100.3 nodes but exactly 100 nodes.
This will make the "center" coordinate more accurate.
Updating the graph during runtime
Any graph which implements the IUpdatableGraph interface can be updated during runtime.
For grid graphs this is a great feature since you can update only a small part of the grid without causing any lag like a complete rescan would.
If you for example just have instantiated a sphere obstacle in the scene and you want to update the grid where that sphere was instantiated, you can do this:
AstarPath.active.UpdateGraphs (ob.collider.bounds);
Where ob is the obstacle you just instantiated (a GameObject).
As you can see, the UpdateGraphs function takes a Bounds parameter and it will send an update call to all updateable graphs.
A grid graph will update that area and a small margin around it equal to collision testing diameter/2
Hexagon graphs
The graph can be configured to work like a hexagon graph with some simple settings. Since 4.1.x the grid graph has a 'Shape' dropdown. If you set it to 'Hexagonal' the graph will behave as a hexagon graph. Often you may want to rotate the graph +45 or -45 degrees. 
Note however that the snapping to the closest node is not exactly as you would expect in a real hexagon graph, but it is close enough that you will likely not notice.
Configure using code
// This holds all graph data
AstarData data = AstarPath.active.data;
// This creates a Grid Graph
GridGraph gg = data.AddGraph(typeof(GridGraph)) as GridGraph;
// Setup a grid graph with some values
int width = 50;
int depth = 50;
float nodeSize = 1;
gg.center = new Vector3(10, 0, 0);
// Updates internal size from the above values
gg.SetDimensions(width, depth, nodeSize);
// Scans all graphs
AstarPath.active.Scan();
Tree colliders
It seems that Unity will only generate tree colliders at runtime when the game is started. For this reason, the grid graph will not pick up tree colliders when outside of play mode but it will pick them up once the game starts. If it still does not pick them up make sure that the trees actually have colliders attached to them and that the tree prefabs are in the correct layer (the layer should be included in the 'Collision Testing' mask).
See
Pathfinding.GraphCollision for documentation on the 'Height Testing' and 'Collision Testing' sections of the grid graph settings.
Inner Types
Public Methods
void
CalculateConnections
(
)
Calculates the grid connections for a single node.
Convenience function, it's slightly faster to use CalculateConnections(int,int) but that will only show when calculating for a large number of nodes. This function will also work for both grid graphs and layered grid graphs.
void
CalculateConnections
(
)
Calculates the grid connections for a single node.
Note that to ensure that connections are completely up to date after updating a node you have to calculate the connections for both the changed node and its neighbours.
In a layered grid graph, this will recalculate the connections for all nodes in the (x,z) cell (it may have multiple layers of nodes).
void
CalculateConnectionsForCellAndNeighbours
(
)
Calculates the grid connections for a cell as well as its neighbours.
This is a useful utility function if you want to modify the walkability of a single node in the graph.
AstarPath.active.AddWorkItem(ctx => {
var grid = AstarPath.active.data.gridGraph;
int x = 5;
int z = 7;
// Mark a single node as unwalkable
grid.GetNode(x, z).Walkable = false;
// Recalculate the connections for that node as well as its neighbours
grid.CalculateConnectionsForCellAndNeighbours(x, z);
});
bool
CheckConnection
(
)
Returns if node is connected to it's neighbour in the specified direction.
This will also return true if neighbours = NumNeighbours.Four, the direction is diagonal and one can move through one of the adjacent nodes to the targeted node.
int
CountNodes
()
Number of nodes in the graph.
Note that this is, unless the graph type has overriden it, an O(n) operation.
This is an O(1) operation for grid graphs and point graphs. For layered grid graphs it is an O(n) operation.
void
ErodeWalkableArea
()
Erodes the walkable area.
void
ErodeWalkableArea
(
int | xmin | |
int | zmin | |
int | xmax | |
int | zmax | |
)
Erodes the walkable area.
xmin, zmin (inclusive)
xmax, zmax (exclusive)
uint
GetConnectionCost
(
)
Vector3 | position | The position to try to find a close node to |
NNConstraint | constraint | Can for example tell the function to try to return a walkable node. If you do not get a good node back, consider calling GetNearestForce. |
GraphNode | hint | Can be passed to enable some graph generators to find the nearest node faster. |
)
Returns the nearest node to a position using the specified NNConstraint.
)
Returns the nearest node to a position using the specified constraint .
Return
an NNInfo. This method will only return an empty NNInfo if there are no nodes which comply with the specified constraint.
)
Node in the specified cell.
Returns null if the coordinate is outside the grid.
var gg = AstarPath.active.data.gridGraph;
int x = 5;
int z = 8;
GridNodeBase node = gg.GetNode(x, z);
If you know the coordinate is inside the grid and you are looking to maximize performance then you can look up the node in the internal array directly which is slightly faster.
void
GetNodes
(
)
Calls a delegate with all nodes in the graph.
This is the primary way of iterating through all nodes in a graph.
Do not change the graph structure inside the delegate.
var gg = AstarPath.active.data.gridGraph;
gg.GetNodes(node => {
// Here is a node
Debug.Log("I found a node at position " + (Vector3)node.position);
});
If you want to store all nodes in a list you can do this
var gg = AstarPath.active.data.gridGraph;
List<GraphNode> nodes = new List<GraphNode>();
gg.GetNodes((System.Action<GraphNode>)nodes.Add);
List<GraphNode>
GetNodesInRegion
(
IntRect | rect | Region in which to return nodes. It will be clamped to the grid. |
)
Get all nodes in a rectangle.
List<GraphNode>
GetNodesInRegion
(
)
All nodes inside the shape.
Note
Be nice to the garbage collector and pool the list when you are done with it (optional)
List<GraphNode>
GetNodesInRegion
(
)
All nodes inside the bounding box.
Note
Be nice to the garbage collector and pool the list when you are done with it (optional)
int
GetNodesInRegion
(
IntRect | rect | Region in which to return nodes. It will be clamped to the grid. |
GridNodeBase[] | buffer | Buffer in which the nodes will be stored. Should be at least as large as the number of nodes that can exist in that region. |
)
Get all nodes in a rectangle.
Return
The number of nodes written to the buffer.
Note
This method is much faster than GetNodesInRegion(IntRect) which returns a list because this method can make use of the highly optimized System.Array.Copy method.
Int3
GraphPointToWorld
(
)
Transform a point in graph space to world space.
This will give you the node position for the node at the given x and z coordinate if it is at the specified height above the base of the graph.
bool
IsValidConnection
(
)
Returns true if a connection between the adjacent nodes n1 and n2 is valid.
Also takes into account if the nodes are walkable.
This method may be overriden if you want to customize what connections are valid. It must however hold that IsValidConnection(a,b) == IsValidConnection(b,a).
This is used for calculating the connections when the graph is scanned or updated.
bool
Linecast
(
)
Returns if there is an obstacle between the two nodes on the graph.
This method is very similar to the other Linecast methods however it is much faster due to being able to use only integer math and not having to look up which node is closest to a particular input point.
var gg = AstarPath.active.data.gridGraph;
var node1 = gg.GetNode(2, 3);
var node2 = gg.GetNode(5, 7);
bool anyObstaclesInTheWay = gg.Linecast(node1, node2);
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
)
Returns if there is an obstacle between from and to on the graph.
This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
var gg = AstarPath.active.data.gridGraph;
bool anyObstaclesInTheWay = gg.Linecast(transform.position, enemy.position);
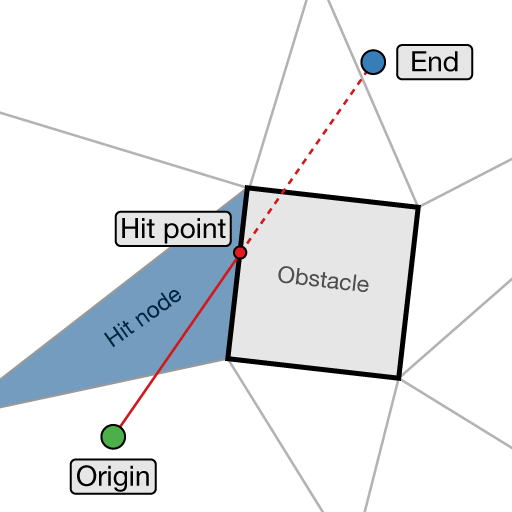
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
Vector3 | from | Point to linecast from |
Vector3 | to | Point to linecast to |
GraphNode | hint | This parameter is deprecated. It will be ignored. |
)
Returns if there is an obstacle between from and to on the graph.
This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
var gg = AstarPath.active.data.gridGraph;
bool anyObstaclesInTheWay = gg.Linecast(transform.position, enemy.position);
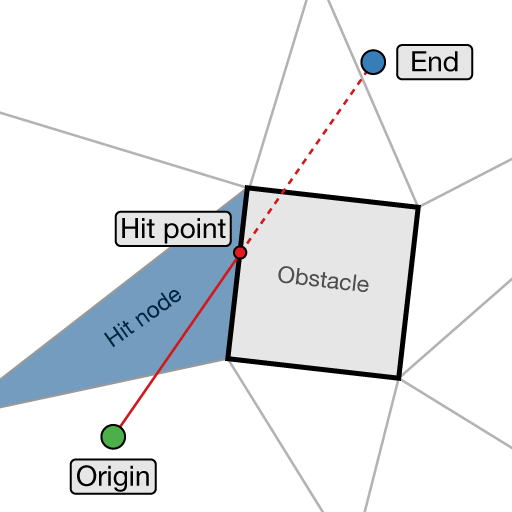
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
)
Returns if there is an obstacle between from and to on the graph.
This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
var gg = AstarPath.active.data.gridGraph;
bool anyObstaclesInTheWay = gg.Linecast(transform.position, enemy.position);
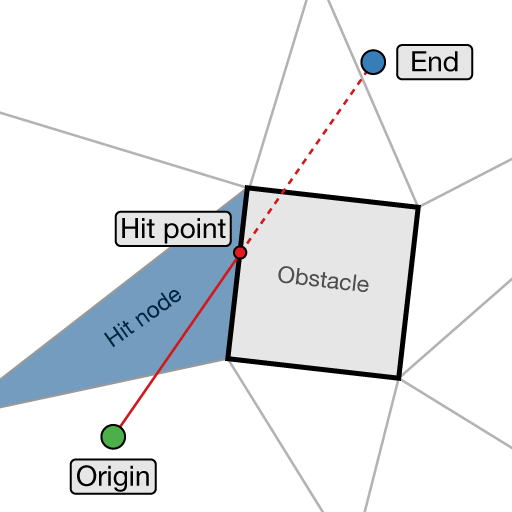
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
bool
Linecast
(
Vector3 | from | Point to linecast from |
Vector3 | to | Point to linecast to |
GraphNode | hint | This parameter is deprecated. It will be ignored. |
outGraphHitInfo | hit | Contains info on what was hit, see GraphHitInfo |
List<GraphNode> | trace | If a list is passed, then it will be filled with all nodes the linecast traverses |
)
Returns if there is an obstacle between from and to on the graph.
This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
var gg = AstarPath.active.data.gridGraph;
bool anyObstaclesInTheWay = gg.Linecast(transform.position, enemy.position);
Version
In 3.6.8 this method was rewritten to improve accuracy and performance. Previously it used a sampling approach which could cut corners of obstacles slightly and was pretty inefficient.
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
void
OnDrawGizmos
(
)
Draw gizmos for the graph.
void
RecalculateCell
(
int | x | X coordinate of the cell |
int | z | Z coordinate of the cell |
bool | resetPenalties=true | If true, the penalty of the nodes will be reset to the initial value as if the graph had just been scanned (this excludes texture data however which is only done when scanning the graph). |
bool | resetTags=true | If true, the tag will be reset to zero (the default tag). |
)
Recalculates single node in the graph.
For a layered grid graph this will recalculate all nodes at a specific (x,z) cell in the grid. For grid graphs this will simply recalculate the single node at those coordinates.
Note
This must only be called when it is safe to update nodes. For example when scanning the graph or during a graph update.
This will not recalculate any connections as this method is often run for several adjacent nodes at a time. After you have recalculated all the nodes you will have to recalculate the connections for the changed nodes as well as their neighbours.
void
RelocateNodes
(
)
Moves the nodes in this graph.
Multiplies all node positions by deltaMatrix.
For example if you want to move all your nodes in e.g a point graph 10 units along the X axis from the initial position var graph = AstarPath.data.pointGraph;
var m = Matrix4x4.TRS (new Vector3(10,0,0), Quaternion.identity, Vector3.one);
graph.RelocateNodes (m);
Note
For grid graphs, navmesh graphs and recast graphs it is recommended to use their custom overloads of the RelocateNodes method which take parameters for e.g center and nodeSize (and additional parameters) instead since they are both easier to use and are less likely to mess up pathfinding.
Warning
This method is lossy for PointGraphs, so calling it many times may cause node positions to lose precision. For example if you set the scale to 0 in one call then all nodes will be scaled/moved to the same point and you will not be able to recover their original positions. The same thing happens for other - less extreme - values as well, but to a lesser degree.
void
RelocateNodes
(
Vector3 | center | |
Quaternion | rotation | |
float | nodeSize | |
float | aspectRatio=1 | |
float | isometricAngle=0 | |
)
Relocate the grid graph using new settings.
This will move all nodes in the graph to new positions which matches the new settings.
void
SetGridShape
(
)
Changes the grid shape.
This is equivalent to changing the 'shape' dropdown in the grid graph inspector.
Calling this method will set isometricAngle, aspectRatio, uniformEdgeCosts and neighbours to appropriate values for that shape.
Note
Setting the shape to InspectorGridMode.Advanced does not do anything except set the inspectorGridMode field.
bool
SnappedLinecast
(
)
Returns if there is an obstacle between from and to on the graph.
This function is different from the other Linecast functions since it snaps the start and end positions to the centers of the closest nodes on the graph. This is not the same as Physics.Linecast, this function traverses the graph and looks for collisions.
Version
Since 3.6.8 this method uses the same implementation as the other linecast methods so there is no performance boost to using it.
In 3.6.8 this method was rewritten and that fixed a large number of bugs. Previously it had not always followed the line exactly as it should have and the hit output was not very accurate (for example the hit point was just the node position instead of a point on the edge which was hit).
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
Public Static Methods
float
ConvertHexagonSizeToNodeSize
(
)
float
ConvertNodeSizeToHexagonSize
(
)
Public Variables
float
aspectRatio = 1F
Scaling of the graph along the X axis.
This should be used if you want different scales on the X and Y axis of the grid
Center point of the grid.
Settings on how to check for walkability and height.
bool
cutCorners = true
If disabled, will not cut corners on obstacles.
If connections is Eight, obstacle corners might be cut by a connection, setting this to false disables that.
int
depth
Depth (height) of the grid in nodes.
int
erodeIterations
Erosion of the graph.
The graph can be eroded after calculation. This means a margin is put around unwalkable nodes or other unwalkable connections. It is really good if your graph contains ledges where the nodes without erosion are walkable too close to the edge.
Below is an image showing a graph with erode iterations 0, 1 and 2 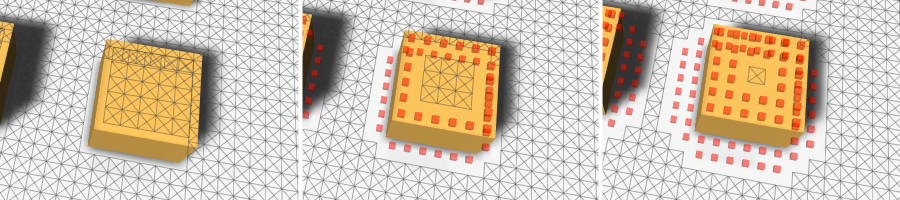
Note
A high number of erode iterations can seriously slow down graph updates during runtime (GraphUpdateObject) and should be kept as low as possible.
int
erosionFirstTag = 1
Tag to start from when using tags for erosion.
const int
getNearestForceOverlap = 2
In GetNearestForce, determines how far to search after a valid node has been found.
InspectorGridMode
inspectorGridMode = InspectorGridMode.Grid
Determines the layout of the grid graph inspector in the Unity Editor.
This field is only used in the editor, it has no effect on the rest of the game whatsoever.
If you want to change the grid shape like in the inspector you can use the SetGridShape method.
InspectorGridHexagonNodeSize
inspectorHexagonSizeMode = InspectorGridHexagonNodeSize.Width
Determines how the size of each hexagon is set in the inspector.
For hexagons the normal nodeSize field doesn't really correspond to anything specific on the hexagon's geometry, so this enum is used to give the user the opportunity to adjust more concrete dimensions of the hexagons without having to pull out a calculator to calculate all the square roots and complicated conversion factors.
This field is only used in the graph inspector, the nodeSize field will always use the same internal units. If you want to set the node size through code then you can use ConvertHexagonSizeToNodeSize.

float
isometricAngle
Angle to use for the isometric projection.
If you are making a 2D isometric game, you may want to use this parameter to adjust the layout of the graph to match your game. This will essentially scale the graph along one of its diagonals to produce something like this:
A perspective view of an isometric graph. 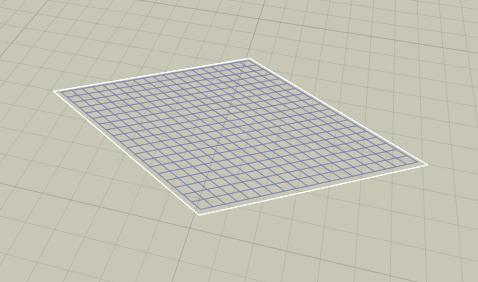
A top down view of an isometric graph. Note that the graph is entirely 2D, there is no perspective in this image. 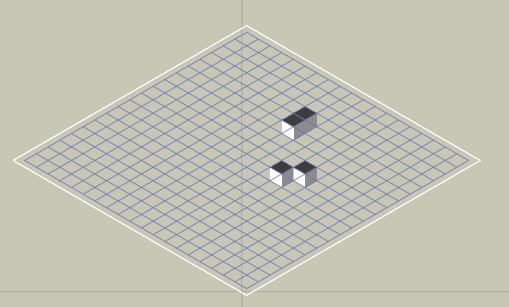
For commonly used values see StandardIsometricAngle and StandardDimetricAngle.
Usually the angle that you want to use is either 30 degrees (alternatively 90-30 = 60 degrees) or atan(1/sqrt(2)) which is approximately 35.264 degrees (alternatively 90 - 35.264 = 54.736 degrees). You might also want to rotate the graph plus or minus 45 degrees around the Y axis to get the oritientation required for your game.
You can read more about it on the wikipedia page linked below.
int
LayerCount
Number of layers in the graph.
For grid graphs this is always 1, for layered grid graphs it can be higher. The nodes array has the size width*depth*layerCount.
float
maxSlope = 90
The max slope in degrees for a node to be walkable.
float
maxStepHeight = 0.4F
The max y coordinate difference between two nodes to enable a connection.
Set to 0 to ignore the value.
This affects for example how the graph is generated around ledges and stairs.
Version
Was previously called maxClimb
bool
maxStepUsesSlope = true
Take the slope into account for maxClimb.
When this is enabled the normals of the terrain will be used to make more accurate estimates of how large the steps are between adjacent nodes.
When this is disabled then calculated step between two nodes is their y coordinate difference. This may be inaccurate, especially at the start of steep slopes.
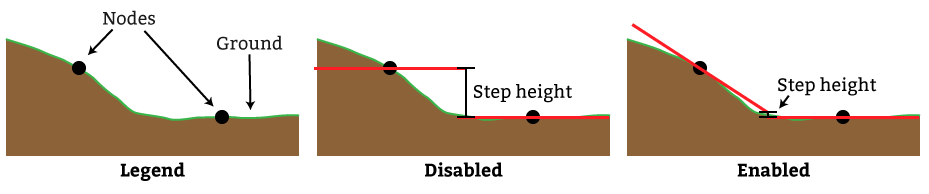
In the image below you can see an example of what happens near a ramp. In the topmost image the ramp is not connected with the rest of the graph which is obviously not what we want. In the middle image an attempt has been made to raise the max step height while keeping maxStepUsesSlope disabled. However this causes too many connections to be added. The agent should not be able to go up the ramp from the side. Finally in the bottommost image the maxStepHeight has been restored to the original value but maxStepUsesSlope has been enabled. This configuration handles the ramp in a much smarter way. Note that all the values in the image are just example values, they may be different for your scene. 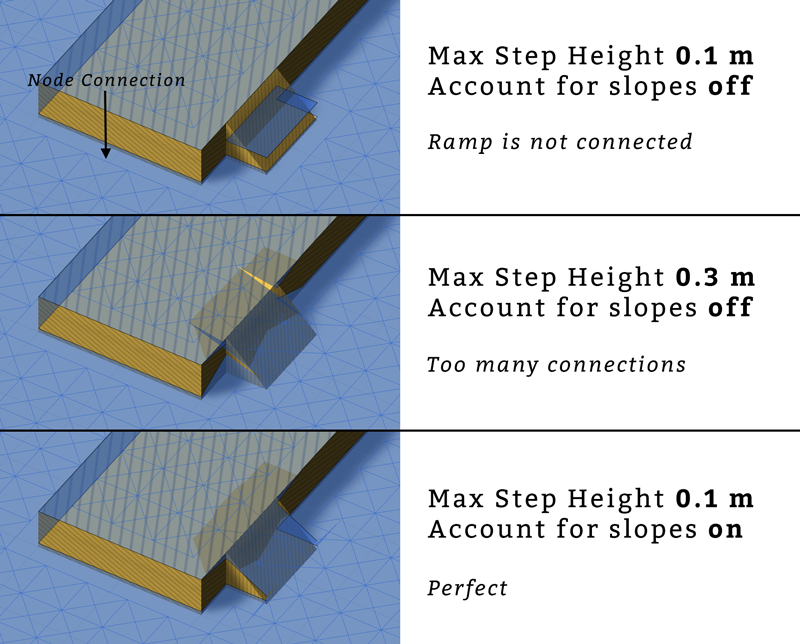
uint []
neighbourCosts = new uint[8]
Costs to neighbour nodes.
int []
neighbourOffsets = new int[8]
Index offset to get neighbour nodes.
Added to a node's index to get a neighbour node index.
Z
|
|
6 2 5
\ | /
-- 3 - X - 1 ----- X
/ | \
7 0 4
|
|
NumNeighbours
neighbours = NumNeighbours.Eight
Number of neighbours for each node.
Either four, six, eight connections per node.
Six connections is primarily for emulating hexagon graphs.
All nodes in this graph.
Nodes are laid out row by row.
The first node has grid coordinates X=0, Z=0, the second one X=1, Z=0
the last one has grid coordinates X=width-1, Z=depth-1.
var gg = AstarPath.active.data.gridGraph;
int x = 5;
int z = 8;
GridNodeBase node = gg.nodes[z*gg.width + x];
float
nodeSize = 1
Size of one node in world units.
Rotation of the grid in degrees.
Additional rules to use when scanning the grid graph.
// Get the first grid graph in the scene
var gridGraph = AstarPath.active.data.gridGraph;
gridGraph.rules.rules.Add(new RuleAnglePenalty {
penaltyScale = 10000,
curve = AnimationCurve.Linear(0, 0, 90, 1),
});
See
#GridGraphRules
#GridGraphRule
bool
showMeshOutline = true
Show an outline of the grid nodes in the Unity Editor.
bool
showMeshSurface = true
Show the surface of the graph.
Each node will be drawn as a square (unless e.g hexagon graph mode has been enabled).
bool
showNodeConnections
Show the connections between the grid nodes in the Unity Editor.
Size of the grid.
Will always be positive and larger than nodeSize.
Size of the grid.
Might be negative or smaller than nodeSize
bool
useJumpPointSearch
Use jump point search to speed up pathfinding.
Jump point search can improve pathfinding performance significantly by making use of some assumptions of the graph. More specifically it assumes a grid graph with no penalties.
int
width
Width of the grid in nodes.
Public Static Variables
int []
neighbourXOffsets = { 0, 1, 0, -1, 1, 1, -1, -1 }
Offsets in the X direction for neighbour nodes.
Only 1, 0 or -1
int []
neighbourZOffsets = { -1, 0, 1, 0, -1, 1, 1, -1 }
Offsets in the Z direction for neighbour nodes.
Only 1, 0 or -1
float
StandardDimetricAngle = Mathf.Acos(1/2f)*Mathf.Rad2Deg
Commonly used value for isometricAngle.
float
StandardIsometricAngle = 90-Mathf.Atan(1/Mathf.Sqrt(2))*Mathf.Rad2Deg
Commonly used value for isometricAngle.
bool
USE_BURST_UPDATE = true
Public Enums
RecalculationMode
RecalculateFromScratch |
Recalculates the nodes from scratch.
Used when the graph is first scanned |
RecalculateMinimal |
Recalculate the minimal number of nodes necessary to guarantee changes inside the graph update's bounding box are taken into account.
Some data may be read from the existing nodes |
NoRecalculation |
Nodes are not recalculated.
Used for graph updates which only set node properties |
Inherited Public Members
Reference to the AstarPath object in the scene.
bool
drawGizmos = true
Enable to draw gizmos in the Unity scene view.
In the inspector this value corresponds to the state of the 'eye' icon in the top left corner of every graph inspector.
Vector3 | position | The position to try to find a close node to |
)
Returns the nearest node to a position.
Vector3 | position | The position to try to find a close node to |
NNConstraint | constraint | Can for example tell the function to try to return a walkable node. If you do not get a good node back, consider calling GetNearestForce. |
)
Returns the nearest node to a position using the specified NNConstraint.
void
GetNodes
(
)
Calls a delegate with all nodes in the graph until the delegate returns false.
uint
graphIndex
Index of the graph, used for identification purposes.
Used as an ID of the graph, considered to be unique.
Note
This is Pathfinding.Util.Guid not System.Guid. A replacement for System.Guid was coded for better compatibility with iOS
bool
infoScreenOpen
Used in the editor to check if the info screen is open.
Should be inside UNITY_EDITOR only #ifs but just in case anyone tries to serialize a NavGraph instance using Unity, I have left it like this as it would otherwise cause a crash when building. Version 3.0.8.1 was released because of this bug only
uint
initialPenalty
Default penalty to apply to all nodes.
string
name
Name of the graph.
Can be set in the unity editor
bool
open
Is the graph open in the editor.
void
Scan
()
Scan the graph.
Consider using AstarPath.Scan() instead since this function only scans this graph and if you are using multiple graphs with connections between them, then it is better to scan all graphs at once.
Private/Protected Members
int | size | |
out JobHandle | dependency | |
)
void
CalculateAffectedRegions
(
)
void
CalculateDimensions
(
out int | width | |
out int | depth | |
out float | nodeSize | |
)
Calculates the width/depth of the graph from unclampedSize and nodeSize.
The node size may be changed due to constraints that the width/depth is not allowed to be larger than 1024 (artificial limit).
bool
ClipLineSegmentToBounds
(
)
Clips a line segment in graph space to the graph bounds.
That is (0,0,0) is the bottom left corner of the graph and (width,0,depth) is the top right corner. The first node is placed at (0.5,y,0.5). One unit distance is the same as nodeSize.
Returns false if the line segment does not intersect the graph at all.
void
CreateNavmeshSurfaceVisualization
(
)
Draw the surface as well as an outline of the grid graph.
The nodes will be drawn as squares (or hexagons when using neighbours = Six).
long
CrossMagnitude
(
)
Magnitude of the cross product a x b.
float
CrossMagnitude
(
)
Magnitude of the cross product a x b.
void
DestroyAllNodes
()
Destroys all nodes in the graph.
Warning
This is an internal method. Unless you have a very good reason, you should probably not call it.
void
DisposeUnmanagedData
()
Cleans up any unmanaged data that the graph has.
Note
The graph has to stay valid after this. However it need not be in a scanned state.
void
DrawUnwalkableNodes
(
)
void
ErodeNode
(
)
Internal method used for erosion.
bool
ErosionAnyFalseConnections
(
)
True if the node has any blocked connections.
For 4 and 8 neighbours the 4 axis aligned connections will be checked. For 6 neighbours all 6 neighbours will be checked.
Internal method used for erosion.
bool
exists
True if the graph exists, false if it has been destroyed.
List<GraphNode>
GetNodesInRegion
(
)
All nodes inside the shape or if null, the bounding box.
If a shape is supplied, it is assumed to be contained inside the bounding box.
IntRect
GetRectFromBounds
(
)
A rect with all nodes that the bounds could touch.
This correctly handles rotated graphs and other transformations. The returned rect is guaranteed to not extend outside the graph bounds.
void
HandleBackwardsCompatibility
(
)
int []
hexagonNeighbourIndices = { 0, 1, 5, 2, 3, 7 }
Which neighbours are going to be used when neighbours=6.
System.Func<GridNodeBase>
newGridNodeDelegate = () => new GridNode()
Delegate which creates and returns a single instance of the node type for this graph.
This may be set in the constructor for graphs inheriting from the GridGraph to change the node type of the graph.
NativeArray<float4>
nodeSurfaceNormals
Surface normal for each node.
This needs to be saved when the maxStepUsesSlope option is enabled for graph updates to work.
void
OnDestroy
()
Function for cleaning up references.
This will be called on the same time as OnDisable on the gameObject which the AstarPath script is attached to (remember, not in the editor). Use for any cleanup code such as cleaning up static variables which otherwise might prevent resources from being collected. Use by creating a function overriding this one in a graph class, but always call base.OnDestroy () in that function. All nodes should be destroyed in this function otherwise a memory leak will arise.
void
PostDeserialization
(
)
Called after all deserialization has been done for all graphs.
Can be used to set up more graph data which is not serialized
void
RemoveGridGraphFromStatic
()
IEnumerable<Progress>
ScanInternal
(
)
Internal method to scan the graph.
Called from AstarPath.ScanAsync. Override this function to implement custom scanning logic. Progress objects can be yielded to show progress info in the editor and to split up processing over several frames when using async scanning.
IEnumerable<Progress>
ScanInternalBurst
(
)
void IUpdatableGraph.
UpdateArea
(
)
Internal function to update an area of the graph.
IEnumerator<JobHandle>
UpdateAreaBurstCoroutine
(
)
void IUpdatableGraph.
UpdateAreaInit
(
)
May be called on the Unity thread before starting the update.
void IUpdatableGraph.
UpdateAreaPost
(
)
May be called on the Unity thread after executing the update.
bool
useRaycastNormal
Use heigh raycasting normal for max slope calculation.
True if maxSlope is less than 90 degrees.
Deprecated Members
void
CalculateConnections
(
)
Calculates the grid connections for a single node.
Deprecated
Use the instance function instead
void
CalculateConnections
(
)
Calculates the grid connections for a single node.
Deprecated
Use CalculateConnections(x,z) or CalculateConnections(node) instead
void
DeserializeSettingsCompatibility
(
)
An old format for serializing settings.
Deprecated
This is deprecated now, but the deserialization code is kept to avoid loosing data when upgrading from older versions.
void
GenerateMatrix
()
Generates the matrix used for translating nodes from grid coordinates to world coordinates.
GridNode
GetNodeConnection
(
)
Deprecated
GridNode
GetNodeConnection
(
int | index | |
int | x | |
int | z | |
int | dir | |
)
Get the connecting node from the node at (x,z) in the specified direction.
Return
A GridNode if the node has a connection to that node. Null if no connection in that direction exists
Deprecated
List<GraphNode>
GetNodesInArea
(
)
Deprecated
This method has been renamed to GetNodesInRegion
List<GraphNode>
GetNodesInArea
(
)
Deprecated
This method has been renamed to GetNodesInRegion
List<GraphNode>
GetNodesInArea
(
)
Deprecated
This method has been renamed to GetNodesInRegion
bool
HasNodeConnection
(
)
Deprecated
bool
HasNodeConnection
(
int | index | |
int | x | |
int | z | |
int | dir | |
)
Deprecated
float
maxClimb
The max y coordinate difference between two nodes to enable a connection.
float
penaltyAngleFactor = 100F
How much penalty is applied depending on the slope of the terrain.
At a 90 degree slope (not that exactly 90 degree slopes can occur, but almost 90 degree), this penalty is applied. At a 45 degree slope, half of this is applied and so on. Note that you may require very large values, a value of 1000 is equivalent to the cost of moving 1 world unit.
float
penaltyAnglePower = 1
How much extra to penalize very steep angles.
bool
penaltyPosition
Use position (y-coordinate) to calculate penalty.
float
penaltyPositionFactor = 1F
Scale factor for penalty when calculating from position.
float
penaltyPositionOffset
Offset for the position when calculating penalty.
void
SetNodeConnection
(
)
Deprecated
void
SetNodeConnection
(
int | index | Index of the node |
int | x | X coordinate of the node |
int | z | Z coordinate of the node |
int | dir | Direction from 0 up to but excluding 8. |
bool | value | Enable or disable the connection |
)
Set if connection in the specified direction should be enabled.
Note that bounds checking will still be done when getting the connection value again, so it is not necessarily true that HasNodeConnection will return true just because you used SetNodeConnection on a node to set a connection to true.
Note
This is identical to Pathfinding.Node.SetConnectionInternal
Deprecated
Holds settings for using a texture as source for a grid graph.
Texure data can be used for fine grained control over how the graph will look. It can be used for positioning, penalty and walkability control.
Below is a screenshot of a grid graph with a penalty map applied. It has the effect of the AI taking the longer path along the green (low penalty) areas.
Color data is got as 0...255 values.
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
Warning
Can only be used with Unity 3.4 and up
void
UpdateNodePositionCollision
(
GridNode | node | |
int | x | |
int | z | |
bool | resetPenalty=true | |
)
Updates position, walkability and penalty for the node.
Assumes that collision.Initialize (...) has been called before this function
Deprecated
Use RecalculateCell instead which works both for grid graphs and layered grid graphs.