void
GetRemainingPath
(
List<Vector3> | buffer | The buffer will be cleared and replaced with the path. The first point is the current position of the agent. |
List<PathPartWithLinkInfo> | partsBuffer | If not null, this list will be filled with information about the different parts of the path. A part is a sequence of nodes or an off-mesh link. |
out bool | stale | May be true if the path is invalid in some way. For example if the agent has no path or if the agent has detected that some nodes in the path have been destroyed. |
)
Fills buffer with the remaining path.
var buffer = new List<Vector3>();
var parts = new List<PathPartWithLinkInfo>();
ai.GetRemainingPath(buffer, parts, out bool stale);
foreach (var part in parts) {
for (int i = part.startIndex; i < part.endIndex; i++) {
Debug.DrawLine(buffer[i], buffer[i+1], part.type == Funnel.PartType.NodeSequence ? Color.red : Color.green);
}
}
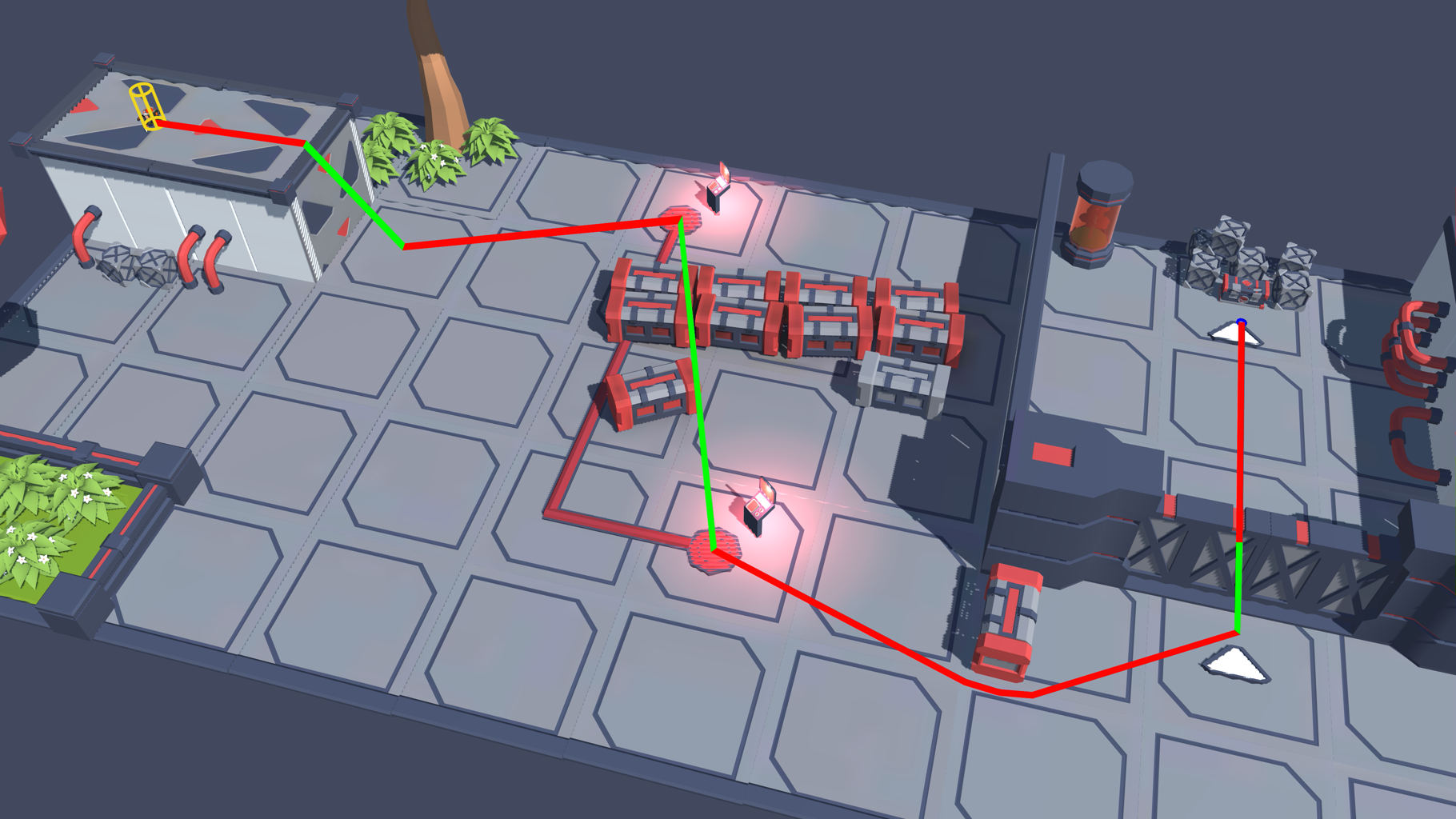
NoteThe FollowerEntity simplifies its path continuously as it moves along it. This means that the agent may not follow this exact path, if it manages to simplify the path later. Furthermore, the agent will apply a steering behavior on top of this path, to make its movement smoother.
void
GetRemainingPath
(
List<Vector3> | buffer | The buffer will be cleared and replaced with the path. The first point is the current position of the agent. |
out bool | stale | May be true if the path is invalid in some way. For example if the agent has no path or if the agent has detected that some nodes in the path have been destroyed. |
)
Fills buffer with the remaining path.
If the agent traverses off-mesh links, the buffer will still contain the whole path. Off-mesh links will be represented by a single line segment. You can use the GetRemainingPath(List<Vector3>,List<PathPartWithLinkInfo>,bool) overload to get more detailed information about the different parts of the path.
var buffer = new List<Vector3>();
ai.GetRemainingPath(buffer, out bool stale);
for (int i = 0; i < buffer.Count - 1; i++) {
Debug.DrawLine(buffer[i], buffer[i+1], Color.red);
}