Class PointGraph Extends NavGraph, IUpdatableGraph
Basic point graph.
The point graph is the most basic graph structure, it consists of a number of interconnected points in space called nodes or waypoints.
The point graph takes a Transform object as "root", this Transform will be searched for child objects, every child object will be treated as a node. If recursive is enabled, it will also search the child objects of the children recursively. It will then check if any connections between the nodes can be made, first it will check if the distance between the nodes isn't too large (maxDistance) and then it will check if the axis aligned distance isn't too high. The axis aligned distance, named limits, is useful because usually an AI cannot climb very high, but linking nodes far away from each other, but on the same Y level should still be possible. limits and maxDistance are treated as being set to infinity if they are set to 0 (zero).
Lastly it will check if there are any obstructions between the nodes using raycasting which can optionally be thick.
One thing to think about when using raycasting is to either place the nodes a small distance above the ground in your scene or to make sure that the ground is not in the raycast mask to avoid the raycast from hitting the ground.
Alternatively, a tag can be used to search for nodes.
For larger graphs, it can take quite some time to scan the graph with the default settings. If you have the pro version you can enable 'optimizeForSparseGraph' which will in most cases reduce the calculation times drastically.
Note
Does not support linecast because of obvious reasons.
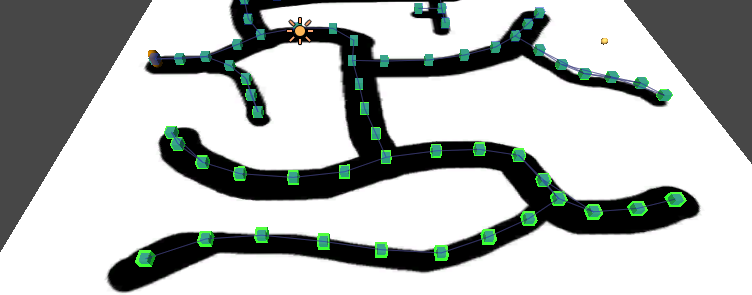
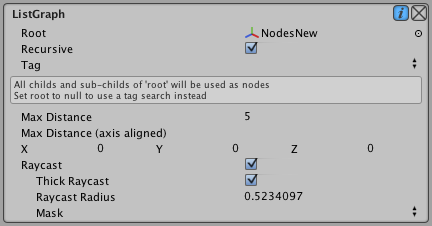
Public Methods
Add a node to the graph at the specified position.
Add a node with the specified type to the graph at the specified position.
Recalculates connections for all nodes in the graph.
Number of nodes in the graph.
Returns the nearest node to a position using the specified NNConstraint.
Returns the nearest node to a position using the specified constraint .
Calls a delegate with all nodes in the graph.
Returns if the connection between a and b is valid.
Rebuilds the lookup structure for nodes.
Moves the nodes in this graph.
Public Variables
Max distance along the axis for a connection to be valid.
Layer mask to use for raycast.
Max distance for a connection to be valid.
Number of nodes in this graph.
All nodes in this graph.
Optimizes the graph for sparse graphs.
Use raycasts to check connections.
Childs of this transform are treated as nodes.
Use thick raycast.
Thick raycast radius.
Use the 2D Physics API.
Inherited Public Members
Enable to draw gizmos in the Unity scene view.
Returns the nearest node to a position.
Returns the nearest node to a position using the specified NNConstraint.
Calls a delegate with all nodes in the graph until the delegate returns false.
Index of the graph, used for identification purposes.
Used as an ID of the graph, considered to be unique.
Used in the editor to check if the info screen is open.
Default penalty to apply to all nodes.
Name of the graph.
Draw gizmos for the graph.
Is the graph open in the editor.
Scan the graph.
Private/Protected Members
Recursively adds childrens of a transform as nodes.
Calculates connections for all nodes in the graph.
Recursively counds children of a transform.
Deserializes graph type specific node data.
Destroys all nodes in the graph.
True if the graph exists, false if it has been destroyed.
Function for cleaning up references.
Called after all deserialization has been done for all graphs.
Internal method to scan the graph.
Serializes graph type specific node data.
Updates an area in the list graph.
Deprecated Members
An old format for serializing settings.