Class GraphUpdateScene Extends GraphModifier
Helper class for easily updating graphs.
The GraphUpdateScene component is really easy to use. Create a new empty GameObject and add the component to it, it can be found in Components–>Pathfinding–>GraphUpdateScene.
When you have added the component, you should see something like the image below.
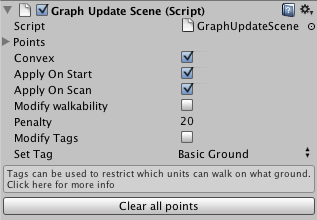
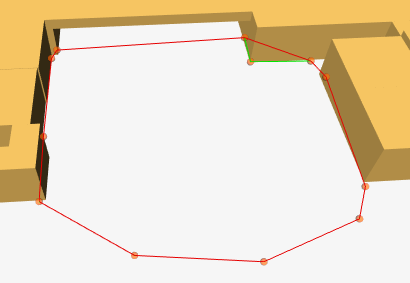
The next two variables, called "Apply On Start" and "Apply On Scan" determine when to apply the changes. If the object is in the scene from the beginning, both can be left on, it doesn't matter since the graph is also scanned at start. However if you instantiate it later in the game, you can make it apply it's setting directly, or wait until the next scan (if any). If the graph is rescanned, all GraphUpdateScene components which have the Apply On Scan variable toggled will apply their settings again to the graph since rescanning clears all previous changes.
You can also make it apply it's changes using scripting.
GetComponent<GraphUpdateScene>().Apply ();
The above code will make it apply its changes to the graph (assuming a GraphUpdateScene component is attached to the same GameObject).Next there is "Modify Walkability" and "Set Walkability" (which appears when "Modify Walkability" is toggled). If Modify Walkability is set, then all nodes inside the area will either be set to walkable or unwalkable depending on the value of the "Set Walkability" variable.
Penalty can also be applied to the nodes. A higher penalty (aka weight) makes the nodes harder to traverse so it will try to avoid those areas.
The tagging variables can be read more about on this page: Working with tags.
Note
The Y (up) axis of the transform that this component is attached to should be in the same direction as the up direction of the graph. So if you for example have a grid in the XY plane then the transform should have the rotation (-90,0,0).
Public Methods
Updates graphs with a created GUO.
Disables legacy mode if it is enabled.
Calculates the bounds for this component.
Inverts all invertable settings for this GUS.
Called right after all graphs have been scanned.
Recalculate convex hull.
Do some stuff at start.
Public Variables
Apply this graph update object whenever a graph is rescanned.
Apply this graph update object on start.
Use the convex hull of the points instead of the original polygon.
Emulates behavior from before version 4.0.
Minumum height of the bounds of the resulting Graph Update Object.
Should the tags of the nodes be modified.
If true, then all affected nodes will be made walkable or unwalkable according to setWalkability.
Penalty to add to nodes.
Points which define the region to update.
Reset penalties to their initial values when updating grid graphs and updatePhysics is true.
Nodes will be made walkable or unwalkable according to this value if modifyWalkability is true.
Update Erosion for GridGraphs.
Update node's walkability and connectivity using physics functions.
Inherited Public Members
Called after graphs have been updated using GraphUpdateObjects or navmesh cutting.
Called after graphs have been updated.
Called before graphs are updated using GraphUpdateObjects.
Called at the end of the scanning procedure.
Called after cached graphs have been loaded.
Called after a graph has been deserialized and loaded.
Called right before graphs are going to be scanned.
Triggers an event for all active graph modifiers.
Private/Protected Members
Has apply been called yet.
Use world space for coordinates.
Removes this modifier from list of active modifiers.
Draws some gizmos.
Draws some gizmos.
Draws some gizmos.
Adds this modifier to list of active modifiers.
Handle serialization backwards compatibility.
Handle serialization backwards compatibility.
Unique persistent ID for this component, used for serialization.
Maps persistent IDs to the component that uses it.
Deprecated Members
Lock all points to a specific Y value.
Switches between using world space and using local space.