Class LayerGridGraph Extends GridGraph, IUpdatableGraph
Grid Graph, supports layered worlds.
The GridGraph is great in many ways, reliable, easily configured and updatable during runtime. But it lacks support for worlds which have multiple layers, such as a building with multiple floors.
That's where this graph type comes in. It supports basically the same stuff as the grid graph, but also multiple layers. It uses a more memory, and is probably a bit slower.
Note
Does not support 8 connections per node, only 4.
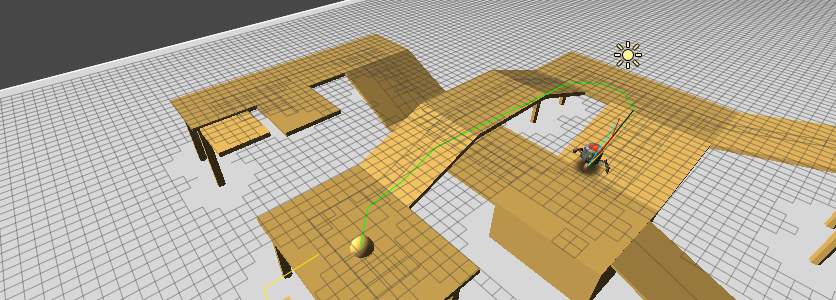
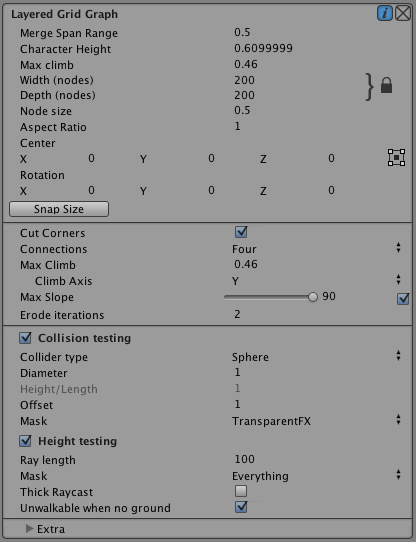
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
Inner Types
Public Methods
Calculates the grid connections for a single node.
Calculates connections for all nodes in a cell (there may be multiple layers of nodes)
Calculates the layered grid graph connections for a single node.
Number of nodes in the graph.
Returns the nearest node to a position using the specified NNConstraint.
Returns the nearest node to a position using the specified constraint .
Node in the specified cell in the first layer.
Node in the specified cell.
Calls a delegate with all nodes in the graph.
Get all nodes in a rectangle.
Get all nodes in a rectangle.
Recalculates single cell.
Public Variables
Nodes with a short distance to the node above it will be set unwalkable.
If two layered nodes are too close, they will be merged.
All nodes in this graph.
Inherited Public Members
Scaling of the graph along the X axis.
Calculates the grid connections for a cell as well as its neighbours.
Returns a new transform which transforms graph space to world space.
Center point of the grid.
Returns if node is connected to it's neighbour in the specified direction.
Settings on how to check for walkability and height.
If disabled, will not cut corners on obstacles.
Depth (height) of the grid in nodes.
Erosion of the graph.
Erodes the walkable area.
Erodes the walkable area.
Tag to start from when using tags for erosion.
Use tags instead of walkability for erosion.
Returns the nearest node to a position.
Returns the nearest node to a position using the specified NNConstraint.
In GetNearestForce, determines how far to search after a valid node has been found.
Calls a delegate with all nodes in the graph until the delegate returns false.
All nodes inside the shape.
All nodes inside the bounding box.
Index of the graph, used for identification purposes.
Transform a point in graph space to world space.
Used as an ID of the graph, considered to be unique.
Used in the editor to check if the info screen is open.
Default penalty to apply to all nodes.
Determines how the size of each hexagon is set in the inspector.
Angle to use for the isometric projection.
Returns true if a connection between the adjacent nodes n1 and n2 is valid.
Number of layers in the graph.
Returns if there is an obstacle between the two nodes on the graph.
Returns if there is an obstacle between from and to on the graph.
Returns if there is an obstacle between from and to on the graph.
Returns if there is an obstacle between from and to on the graph.
Returns if there is an obstacle between from and to on the graph.
The max slope in degrees for a node to be walkable.
The max y coordinate difference between two nodes to enable a connection.
Name of the graph.
Costs to neighbour nodes.
Index offset to get neighbour nodes.
Number of neighbours for each node.
Offsets in the X direction for neighbour nodes.
Offsets in the Z direction for neighbour nodes.
All nodes in this graph.
Size of one node in world units.
Draw gizmos for the graph.
Is the graph open in the editor.
How much penalty is applied depending on the slope of the terrain.
How much extra to penalize very steep angles.
Use position (y-coordinate) to calculate penalty.
Scale factor for penalty when calculating from position.
Offset for the position when calculating penalty.
Moves the nodes in this graph.
Relocate the grid graph using new settings.
Rotation of the grid in degrees.
Scan the graph.
Updates unclampedSize from width, depth and nodeSize values.
Show the surface of the graph.
Size of the grid.
Returns if there is an obstacle between from and to on the graph.
Holds settings for using a texture as source for a grid graph.
Determines how the graph transforms graph space to world space.
Size of the grid.
If true, all edge costs will be set to the same value.
This is placed here so generators inheriting from this one can override it and set it to false.
Use jump point search to speed up pathfinding.
Width of the grid in nodes.
Private/Protected Members
Increases the capacity of the nodes array to hold more layers.
Clips a line segment in graph space to the graph bounds.
Sorts RaycastHits by distance.
Magnitude of the cross product a x b.
Magnitude of the cross product a x b.
Deserializes graph type specific node data.
Destroys all nodes in the graph.
True if the node has any blocked connections.
True if the graph exists, false if it has been destroyed.
All nodes inside the shape or if null, the bounding box.
A rect with all nodes that the bounds could touch.
Which neighbours are going to be used when neighbours=6.
Number of layers.
This function will be called when this graph is destroyed.
Called after all deserialization has been done for all graphs.
Fires a ray from the sky and returns a sample for everything it hits.
Internal method to scan the graph.
Serializes graph type specific node data.
Updates an area using the specified #GraphUpdateObject.
Use heigh raycasting normal for max slope calculation.
Deprecated Members
Calculates the layered grid graph connections for a single node.
Returns if node is connected to it's neighbour in the specified direction.
Internal method to scan the graph.