Class ConstantPath
Extends
Path
Public
Finds all nodes within a specified distance from the start.
This class will search outwards from the start point and find all nodes which it costs less than ConstantPath.maxGScore to reach, this is usually the same as the distance to them multiplied with 1000
The path can be called like: // Here you create a new path and set how far it should search. Null is for the callback, but the seeker will handle that
ConstantPath cpath = ConstantPath.Construct(transform.position, 2000, null);
// Set the seeker to search for the path (where mySeeker is a variable referencing a Seeker component)
mySeeker.StartPath(cpath, myCallbackFunction);
Then when getting the callback, all nodes will be stored in the variable ConstantPath.allNodes (remember that you need to cast it from Path to ConstantPath first to get the variable).
This list will be sorted by the cost to reach that node (more specifically the G score if you are familiar with the terminology for search algorithms). 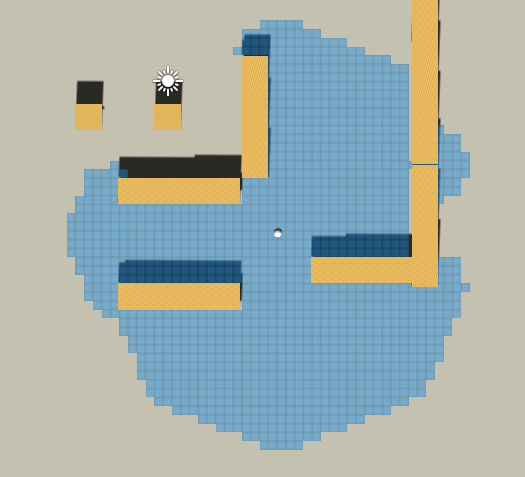
A* Pro Feature:
This is an A* Pathfinding Project Pro feature only. This function/class/variable might not exist in the Free version of the A* Pathfinding Project or the functionality might be limited.
The Pro version can be bought here
Public Static Methods
Vector3 | start | From where the path will be started from (the closest node to that point will be used) |
int | maxGScore | Searching will be stopped when a node has a G score greater than this |
OnPathDelegate | callback=null | Will be called when the path has completed, leave this to null if you use a Seeker to handle calls |
)
Constructs a ConstantPath starting from the specified point.
Searching will be stopped when a node has a G score (cost to reach it) greater or equal to maxGScore in order words it will search all nodes with a cost to get there less than maxGScore.
Public Variables
Contains all nodes the path found.
This list will be sorted by G score (cost/distance to reach the node).
Controls when the path should terminate.
This is set up automatically in the constructor to an instance of the Pathfinding.EndingConditionDistance class with a maxGScore is specified in the constructor. If you want to use another ending condition.
Inherited Public Members
void
BlockUntilCalculated
()
Blocks until this path has been calculated and returned.
Normally it takes a few frames for a path to be calculated and returned. This function will ensure that the path will be calculated when this function returns and that the callback for that path has been called.
Use this function only if you really need to. There is a point to spreading path calculations out over several frames. It smoothes out the framerate and makes sure requesting a large number of paths at the same time does not cause lag.
Note
Graph updates and other callbacks might get called during the execution of this function.
Path p = seeker.StartPath (transform.position, transform.position + Vector3.forward * 10);
p.BlockUntilCalculated();
// The path is calculated now
Callback to call when the path is complete.
This is usually sent to the Seeker component which post processes the path and then calls a callback to the script which requested the path
bool
CanTraverse
(
)
Returns if the node can be traversed.
This by default equals to if the node is walkable and if the node's tag is included in enabledTags.
bool
CanTraverse
(
)
Returns if the path can traverse a link between from and to and if to can be traversed itself.
This by default equals to if the to is walkable and if the to's tag is included in enabledTags.
void
Claim
(
)
Claim this path (pooling).
A claim on a path will ensure that it is not pooled. If you are using a path, you will want to claim it when you first get it and then release it when you will not use it anymore. When there are no claims on the path, it will be reset and put in a pool.
This is essentially just reference counting.
The object passed to this method is merely used as a way to more easily detect when pooling is not done correctly. It can be any object, when used from a movement script you can just pass "this". This class will throw an exception if you try to call Claim on the same path twice with the same object (which is usually not what you want) or if you try to call Release with an object that has not been used in a Claim call for that path. The object passed to the Claim method needs to be the same as the one you pass to this method.
Current state of the path.
float
duration
How long it took to calculate this path in milliseconds.
bool
error
If the path failed, this is true.
See
errorLog
This is equivalent to checking path.CompleteState == PathCompleteState.Error
void
Error
()
Aborts the path because of an error.
Sets error to true. This function is called when an error has occurred (e.g a valid path could not be found).
string
errorLog
Additional info on why a path failed.
void
FailWithError
(
)
Causes the path to fail and sets errorLog to msg.
bool
FloodingPath
True for paths that want to search all nodes and not jump over nodes as optimizations.
This disables Jump Point Search when that is enabled to prevent e.g ConstantPath and FloodPath to become completely useless.
uint
GetConnectionSpecialCost
(
GraphNode | a | Moving from this node |
GraphNode | b | Moving to this node |
uint | currentCost | The cost of moving between the nodes. Return this value if there is no meaningful special cost to return. |
)
May be called by graph nodes to get a special cost for some connections.
Nodes may call it when PathNode.flag2 is set to true, for example mesh nodes, which have a very large area can be marked on the start and end nodes, this method will be called to get the actual cost for moving from the start position to its neighbours instead of as would otherwise be the case, from the start node's position to its neighbours. The position of a node and the actual start point on the node can vary quite a lot.
The default behaviour of this method is to return the previous cost of the connection, essentiall making no change at all.
This method should return the same regardless of the order of a and b. That is f(a,b) == f(b,a) should hold.
State of the path in the pathfinding pipeline.
uint
GetTagPenalty
(
int | tag | A value between 0 (inclusive) and 32 (exclusive). |
)
Returns penalty for the given tag.
float
GetTotalLength
()
Total Length of the path.
Calculates the total length of the vectorPath. Cache this rather than call this function every time since it will calculate the length every time, not just return a cached value.
uint
GetTraversalCost
(
)
Returns the cost of traversing the given node.
Determines which heuristic to use.
float
heuristicScale = 1F
Scale of the heuristic values.
bool
IsDone
()
True if this path is done calculating.
Note
The callback for the path might not have been called yet.
See
Seeker.IsDone which also takes into account if the path callback has been called and had Modifiers applied.
Constraint for how to search for nodes.
Holds the path as a Node array.
All nodes the path traverses. This may not be the same nodes as the post processed path traverses.
ushort
pathID
ID of this path.
Used to distinguish between different paths
Returns the state of the path in the pathfinding pipeline.
void
Release
(
System.Object | o | |
bool | silent=false | |
)
Releases a path claim (pooling).
Removes the claim of the path by the specified object. When the claim count reaches zero, the path will be pooled, all variables will be cleared and the path will be put in a pool to be used again. This is great for performance since fewer allocations are made.
If the silent parameter is true, this method will remove the claim by the specified object but the path will not be pooled if the claim count reches zero unless a Release call (not silent) has been made earlier. This is used by the internal pathfinding components such as Seeker and AstarPath so that they will not cause paths to be pooled. This enables users to skip the claim/release calls if they want without the path being pooled by the Seeker or AstarPath and thus causing strange bugs.
int
searchedNodes
Number of nodes this path has searched.
int[]
tagPenalties
Penalties for each tag.
Tag 0 which is the default tag, will have added a penalty of tagPenalties[0]. These should only be positive values since the A* algorithm cannot handle negative penalties.
When assigning an array to this property it must have a length of 32.
Note
Setting this to null, or trying to assign an array which does not have a length of 32, will make all tag penalties be treated as if they are zero.
If you are using a Seeker. The Seeker will set this value to what is set in the inspector field when you call seeker.StartPath. So you need to change the Seeker's value via script, not set this value.
Provides additional traversal information to a path request.
List<Vector3>
vectorPath
Holds the (possibly post processed) path as a Vector3 list.
IEnumerator
WaitForPath
()
Waits until this path has been calculated and returned.
Allows for very easy scripting. IEnumerator Start () {
var path = seeker.StartPath(transform.position, transform.position + transform.forward*10, null);
yield return StartCoroutine(path.WaitForPath());
// The path is calculated now
}
Private/Protected Members
uint
CalculateHScore
(
)
Estimated cost from the specified node to the target.
void
CalculateStep
(
)
Calculates the until it is complete or the time has progressed past targetTick.
void
Cleanup
()
Always called after the path has been calculated.
Guaranteed to be called before other paths have been calculated on the same thread. Use for cleaning up things like node tagging and similar.
The node currently being processed.
string
DebugString
(
)
Returns a string with information about it.
More information is emitted when logMode == Heavy. An empty string is returned if logMode == None or logMode == OnlyErrors and this path did not fail.
void
DebugStringPrefix
(
PathLog | logMode | |
System.Text.StringBuilder | text | |
)
Writes text shared for all overrides of DebugString to the string builder.
void
DebugStringSuffix
(
PathLog | logMode | |
System.Text.StringBuilder | text | |
)
Writes text shared for all overrides of DebugString to the string builder.
bool
hasBeenReset
True if the Reset function has been called.
Used to alert users when they are doing something wrong.
Target to use for H score calculations.
Target to use for H score calculation.
Used alongside hTarget.
void
Initialize
()
Initializes the path.
Sets up the open list and adds the first node to it
int[]
internalTagPenalties
The tag penalties that are actually used.
If manualTagPenalties is null, this will be ZeroTagPenalties
void
Log
(
)
Appends a message to the errorLog.
Nothing is logged to the console.
Note
If AstarPath.logPathResults is PathLog.None and this is a standalone player, nothing will be logged as an optimization.
void
LogError
(
)
Logs an error.
int[]
manualTagPenalties
Tag penalties set by other scripts.
Internal linked list implementation.
Warning
This is used internally by the system. You should never change this.
void
OnEnterPool
()
Called when the path enters the pool.
This method should release e.g pooled lists and other pooled resources The base version of this method releases vectorPath and path lists. Reset() will be called after this function, not before.
Warning
Do not call this function manually.
Data for the thread calculating this path.
void
Prepare
()
Called before the path is started.
Called right before Initialize
void
PrepareBase
(
)
Prepares low level path variables for calculation.
Called before a path search will take place. Always called before the Prepare, Initialize and CalculateStep functions
bool
recycled
True if the path is currently recycled (i.e in the path pool).
Do not set this value. Only read. It is used internally.
void
ReleaseSilent
(
)
Releases the path silently (pooling).
void
Reset
()
Reset the path to default values.
Clears the allNodes list.
Also sets heuristic to Heuristic.None as it is the default value for this path type
void
ReturnPath
()
Calls callback to return the calculated path.
void
Setup
(
)
Sets up a ConstantPath starting from the specified point.
void
Trace
(
)
Traces the calculated path from the end node to the start.
This will build an array (path) of the nodes this path will pass through and also set the vectorPath array to the path arrays positions. Assumes the vectorPath and path are empty and not null (which will be the case for a correctly initialized path).
int[]
ZeroTagPenalties = new int[32]
List of zeroes to use as default tag penalties.