Class AILerp Extends VersionedMonoBehaviour, IAstarAI
Linearly interpolating movement script.
This movement script will follow the path exactly, it uses linear interpolation to move between the waypoints in the path. This is desirable for some types of games. It also works in 2D.
See
You can see an example of this script in action in the example scene called Example15_2D.
Configuration
Recommended setup for movement along connections
This depends on what type of movement you are aiming for. If you are aiming for movement where the unit follows the path exactly and move only along the graph connections on a grid/point graph. I recommend that you adjust the StartEndModifier on the Seeker component: set the 'Start Point Snapping' field to 'NodeConnection' and the 'End Point Snapping' field to 'SnapToNode'.
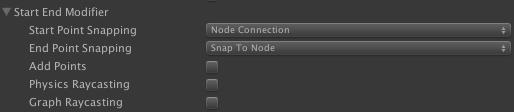
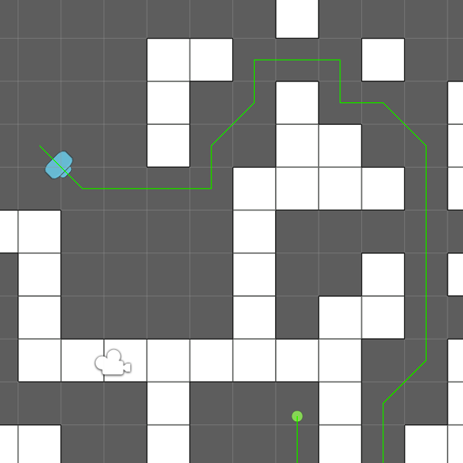
Recommended setup for smooth movement
If you on the other hand want smoother movement I recommend setting 'Start Point Snapping' and 'End Point Snapping' to 'ClosestOnNode' and to add the Simple Smooth Modifier to the GameObject as well. Alternatively you can use the Funnel Modifier which works better on navmesh/recast graphs or the RaycastModifier.
You should not combine the Simple Smooth Modifier or the Funnel Modifier with the NodeConnection snapping mode. This may lead to very odd behavior.
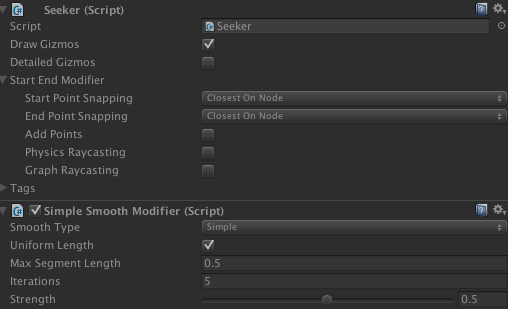
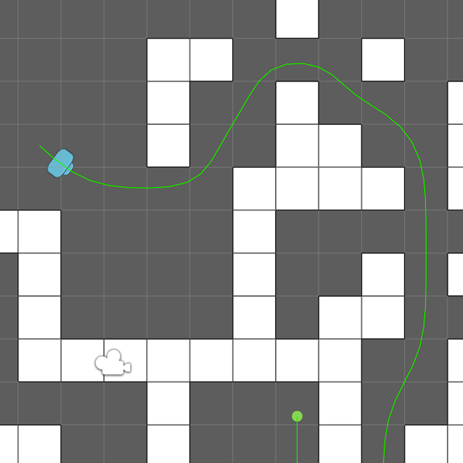
Public Methods
Move the agent.
Calculate how the character wants to move during this frame.
The end of the path has been reached.
Requests a path to the target.
Make the AI follow the specified path.
Instantly move the agent to a new position.
Public Variables
Enables or disables movement completely.
Enables or disables recalculating the path at regular intervals.
If true, the AI will rotate to face the movement direction.
True if this agent currently has a path that it follows.
If true, some interpolation will be done when a new path has been calculated.
Gets or sets if the agent should stop moving.
Called when the agent recalculates its path.
Determines which direction the agent moves in.
True if a path is currently being calculated.
Position of the agent.
True if the end of the current path has been reached.
Remaining distance along the current path to the end of the path.
Determines how often it will search for new paths.
Rotation of the agent.
How quickly to rotate.
Speed in world units.
How quickly to interpolate to the new path.
Determines if the character's position should be coupled to the Transform's position.
Determines if the character's rotation should be coupled to the Transform's rotation.
Private/Protected Members
Initializes reference variables.
Calculate the AI's next position (one frame in the future).
Enables or disables movement completely.
Enables or disables recalculating the path at regular intervals.
Only when the previous path has been returned should a search for a new path be done.
Finds the closest point on the current path and configures the interpolator.
Radius of the agent in world units.
Time when the last path request was sent.
Max speed in world units per second.
Move the agent.
Called when the component is enabled.
Called when a requested path has finished calculation.
Handle serialization backwards compatibility.
Current path which is followed.
Time since the path was replaced by a new path.
When a new path was returned, the AI was moving along this ray.
Height of the agent in world units.
Handle serialization backwards compatibility.
True if the path should be automatically recalculated as soon as possible.
Starts searching for paths.
Holds if the Start function has been run.
Point on the path which the agent is currently moving towards.
Required for serialization backward compatibility.
Cached Transform component.
Deprecated Members
Requests a path to the target.
If true, the forward axis of the character will be along the Y axis instead of the Z axis.
Target to move towards.